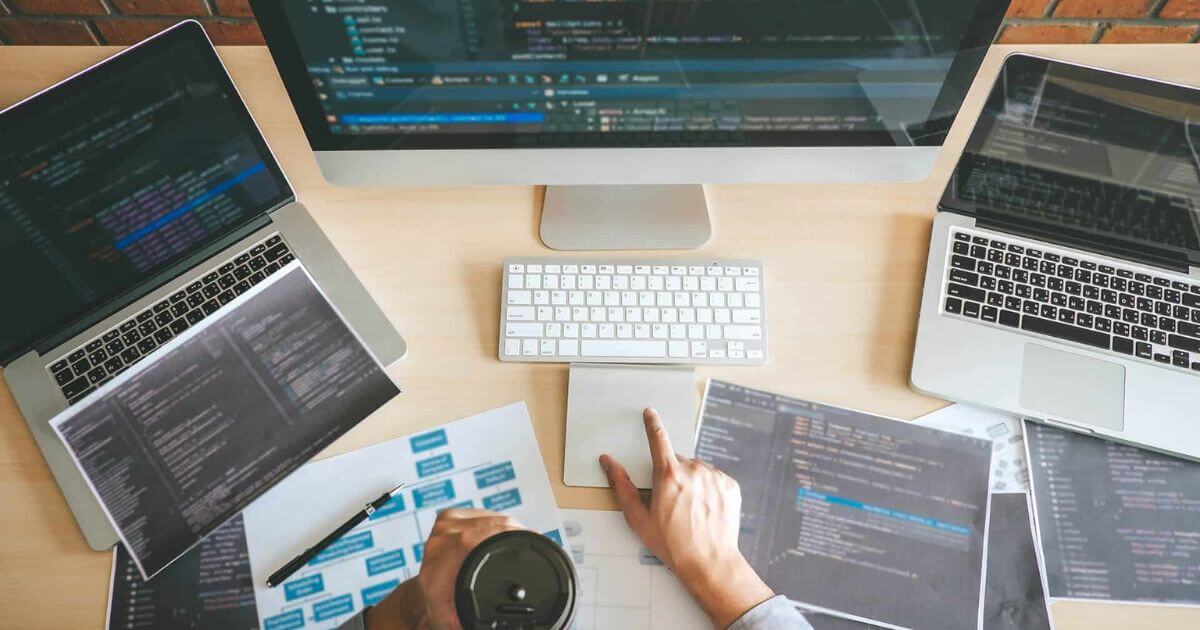
URL Parser
URL Parser
When you're traversing the complexities of web development, understanding how a URL parser operates can greatly enhance your approach. It breaks down URLs into digestible parts, enabling efficient data manipulation that ultimately improves security and user experience. But what does this mean for your projects? As you explore further, you'll discover not just the mechanics behind URL parsing, but also the compelling benefits it brings to your applications—and how it can transform your coding practices in ways you might not expect.
Understanding URL Structure
When you break down a URL, you'll find it consists of several key components that serve distinct purposes. The first part is the protocol, often "http" or "https." This indicates how your browser should fetch the resource from the server. If it's "https," you can feel reassured that your connection is secured with encryption.
Next, you've got the web address, which is the human-readable address of the website. This could be something like "example.com." The web address points to the specific server where the website resides.
Then comes the path, indicating the specific resource you want to access on that server. For instance, "example.com/page" directs you to a particular page.
After the path, you may notice a question mark, signifying the beginning of query parameters. These provide additional data to the server. For example, "id=123" could retrieve a specific item from a database.
How URL Parsers Work
URL parsers are essential tools that break down a given URL into its individual components. When you input a URL, the parser analyzes its structure and extracts key elements like the scheme, host, port, path, query string, and fragment. You can think of it as a translator that deciphers the format and structure of the URL.
First, the parser identifies the scheme (e.g., HTTP or HTTPS) by looking for the specific prefix. Next, it locates the host, which indicates where the resource resides. If the URL includes a port number, the parser captures that too.
Then, the parser examines the path, which directs to the specific resource on the server. After that, it parses the query string, a section that often includes parameters and values, essential for retrieving dynamic content.
Benefits of Using URL Parsers
Using a URL parser can greatly enhance your web development and data handling processes. It allows you to efficiently dissect URLs into their components, such as the protocol, web address, path, and query strings. This breakdown aids in managing web requests and responses more effectively, saving time and reducing errors.
One of the main benefits is improved data extraction. With a URL parser, you can programmatically retrieve specific information from URLs, which is invaluable when dealing with dynamic content. You won't have to manually sift through strings to find the data you need.
Additionally, URL parsers streamline your code. Instead of writing repetitive and complex string manipulation routines, you can use built-in functions to handle URL parsing. This not only makes your code cleaner but also enhances maintainability, as any changes to URL handling can be made in one place.
Moreover, they improve security. By precisely parsing URLs, you can validate input from users and mitigate risks such as injection attacks.
Common Use Cases
Developers frequently encounter scenarios where URL parsing proves invaluable. For instance, when you're building web applications, extracting query parameters from URLs can help you tailor the user experience. If your app retrieves different content based on a user's selections, parsing the URL allows you to dynamically load the right data.
Another common use case is authentication. When users log in to your application, you might use tokens embedded in the URL for secure access. Parsing those tokens is essential for validating user sessions and ensuring security measures in place are effective.
Additionally, when managing links for SEO, you might need to analyze various aspects of URLs to optimize your website. Extracting certain components like paths or parameters can help you identify trends in user behavior or pinpoint issues that need addressing.
You may also find URL parsing helpful for handling redirects. Triggering specific actions based on parsed segments can improve how your application responds to users, directing them to the intended content efficiently.
Popular URL Parsing Libraries
When it comes to efficiently parsing URLs, several libraries stand out for their ease of use and robust features.
If you're working in Python, 'urllib.parse' is a built-in library that's simple yet powerful for handling URLs. It allows you to split URLs into their components or build new ones.
For JavaScript developers, you can't go wrong with 'URL' and 'URLSearchParams', which are part of the standard Web API. They let you manipulate URLs seamlessly in the browser and Node.js, making it a go-to choice for front-end tasks.
In the Java world, 'java.net.URI' is a solid option for parsing and constructing URLs, providing a clean interface. If you're looking for something with more functionality, libraries like 'jsoup' can scrape web data and parse URLs.
For Ruby enthusiasts, 'URI' is built into the standard library and offers a straightforward approach to URL handling.
Finally, for C#, 'System.Uri' provides extensive methods for parsing and working with URLs efficiently.
Each of these libraries enhances your ability to manage URLs, so pick one that fits your project requirements and get started!
Conclusion
To conclude, using a URL parser can greatly streamline your web development process. For instance, did you know that over 70% of web developers rely on URL parsing libraries to enhance their applications? By dissecting URLs into essential components, you're not just improving security and user experience but also increasing your code's maintainability. Adopting these tools can empower you to create more dynamic, user-friendly applications without the hassle of manually handling every URL detail.